
[ad_1]
Introduction
Python is a flexible programming language that gives builders numerous functionalities. One widespread process that Python builders typically encounter is eradicating duplicates from a listing. This weblog will discover 7 methods to take away duplicates from a listing, together with code examples and explanations.
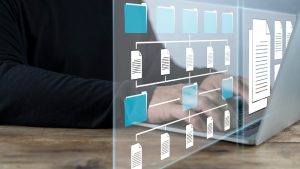
Studying Goals
- Study concerning the traits of Python lists, reminiscent of being ordered, mutable, and capable of maintain any knowledge kind.
- Perceive the essential operations on lists, together with creation, entry by index, modification, addition, and removing of components.
- Acquire hands-on expertise by means of code examples and explanations for every approach, together with set(), record comprehension, filter(), OrderedDict, Counter, itertools.groupby(), and pandas’ drop_duplicates().
What’s a Record?
You may retailer a number of values in a single variable utilizing lists, as they’re ordered collections of things.
Key traits of a Record:
- Ordered: Gadgets preserve a particular order, accessible by their index.
- Mutable: After creating the record, you may change, add, or take away gadgets.
- Can maintain any knowledge kind: Lists can comprise blended knowledge varieties (numbers, strings, different lists, and so forth.)
- Created utilizing sq. brackets []
Examples:
# Creating a listing
furnitures = ["chair", "table", "sofa"]
# Accessing components by index (begins from 0)
first_furniture = furnitures[0]
print(first_furniture)
Output:
"chair"
last_furniture = furnishings[-1] #(detrimental index begins from the top)
print(last_furniture)
Output:
"cherry"
# Modifying components
furnitures[1] = "mattress" # Substitute "desk" with "mattress"
# Including components
furnitures.append("mattress") # Add "mattress" to the top
furnitures.insert(2, "desk") # Insert "desk" at index 2
# Eradicating components
furnitures.take away("mattress") # Take away the primary incidence of "cherry"
del furnitures[0] # Delete the primary aspect
# Different widespread operations
furnitures.pop() # Take away and return the final aspect
furnitures.index("mattress") # Discover the index of "mattress"
furnitures.depend("desk") # Rely the occurrences of "desk"
furnitures.type() # Type the record alphabetically
furnitures.reverse() # Reverse the order of the record
Additionally Learn: Introduction to Python Programming (Newbie’s Information)
Utilizing Set to Take away Duplicates
The primary technique to take away duplicates from a listing is utilizing Python’s set() perform. This technique is easy and environment friendly. Property don’t permit duplicate components.
Convert the Record to a Set
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_set = set(my_list)
print(unique_set)
Output:
{1, 2, 3, 4, 5}
- When you want a listing construction once more, convert the set again to a listing (elective):
unique_list = record(unique_set)
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Key factors:
- Order will not be preserved: Units are unordered, so the ensuing record’s aspect order would possibly differ from the unique.
- Effectivity: Units are optimized for quick membership checks and duplicate removing, making this a really environment friendly technique.
Utilizing Record Comprehension
Record comprehension is a robust function in Python that permits us to create new lists concisely and readably. We are able to leverage record comprehension to take away duplicates from a listing.
Create a New Record with Distinctive Parts
- Use a listing comprehension that iterates by means of the unique record and provides every distinctive aspect to a brand new record.
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = [x for x in my_list if x not in unique_list]
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Rationalization:
- x for x in my_list: Iterates by means of every aspect x within the authentic record.
- if x will not be in unique_list: Checks if the present aspect x is already within the new record. If not, it’s added to the brand new record.
- This creates a listing with distinctive components, preserving their authentic order.
Key factors:
- Order is preserved: Not like utilizing units, record comprehension maintains the unique order of components.
- Effectivity: Record comprehensions could be environment friendly for smaller lists.
- Readability: The code is concise and infrequently thought of extra readable than utilizing units.
Utilizing the Filter() Operate
Python’s filter() perform can be utilized to create a brand new record with components that fulfill a sure situation. We are able to use the filter() perform to take away duplicates from a listing.
Outline a Filtering Operate
- Create a perform that checks if a component has but to be seen earlier than.
def is_unique(x, seen):
return x not in seen
Apply Filter() with a Set to Observe Distinctive Parts
- Use filter() to use every aspect’s is_unique() perform.
- Keep a set to trace seen components for environment friendly membership checks.
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = record(filter(lambda x: is_unique(x, set()), my_list))
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Rationalization:
- filter() takes a perform and an iterable (the record).
- It calls the perform for every aspect, retaining solely these the place the perform returns True.
- lambda x: is_unique(x, set()): Creates a brief nameless perform that calls is_unique() for every aspect.
- set() is used inside is_unique() to effectively examine if a component is already within the seen set.
Key factors:
- Order is preserved: Like record comprehension, filter() maintains the unique order.
- Flexibility: filter() can be utilized with different logic as wanted.
- Effectivity: Whereas typically much less environment friendly than units or record comprehensions, it provides flexibility for customized filtering.
Utilizing the OrderedDict Class
The OrderedDict class in Python is a dict subclass that remembers the order by which gadgets are added. We are able to use the OrderedDict class to take away duplicates from a listing whereas preserving the unique order of components.
- Import the OrderedDict class from collections import OrderedDict
- Create an OrderedDict from the record: This routinely removes duplicates primarily based on keys, preserving order:
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_dict = OrderedDict.fromkeys(my_list)
- Extract the keys as a listing:
unique_list = record(unique_dict.keys())
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Key factors:
- Order is preserved: OrderedDict maintains the unique order of components.
- Optimized for insertions: It’s extra environment friendly than common dictionaries for frequent insertions at the start or finish.
- Distinctive keys: Dictionary keys have to be distinctive, so duplicates are routinely eliminated.
Utilizing the Counter Class
The Counter class in Python is a robust software for counting the occurrences of components in a listing. We are able to leverage the Counter class to take away duplicates from a listing and procure the depend of every distinctive aspect.
- Import the Counter class from collections import Counter
- Create a Counter object from the record: The counter counts the occurrences of every aspect:
my_list = [1, 2, 2, 3, 4, 4, 5]
counter = Counter(my_list)
- Extract the distinctive components: Use the keys() technique to get a listing of distinctive components:
unique_list = record(counter.keys())
print(unique_list)
Output:
[1, 2, 3, 4, 5]
Key factors:
- Order will not be assured: Counter doesn’t protect the unique order.
- Ingredient counts: It offers aspect frequencies if wanted:
print(counter.most_common())
Output:
[(2, 2), (4, 2), (1, 1), (3, 1), (5, 1)]
The itertools module in Python offers a groupby() perform that permits us to group components primarily based on a key perform. We are able to use the groupby() perform to take away duplicates from a listing.
- Import the groupby() perform: from itertools import groupby
- Type the record: groupby() works on consecutive components, so type the record first:
my_list = [1, 2, 2, 3, 4, 4, 5]
my_list.type() # [1, 2, 2, 3, 4, 4, 5]
- Apply groupby() and extract distinctive components: Group consecutive components collectively:
unique_elements = [key for key, _ in groupby(my_list)]
print(unique_elements)
Output:
[1, 2, 3, 4, 5]
Rationalization:
- groupby() takes an iterable (just like the sorted record) and a key perform (defaults to the identification perform).
- It returns an iterator that yields consecutive keys and teams of components.
- key for key, _ in groupby(…): Makes use of a generator expression to extract solely the keys (distinctive components) from the teams.
Key factors:
- Order is preserved: Sorting maintains the unique order of distinctive components.
- Effectivity: groupby() could be environment friendly for bigger lists, because it avoids creating intermediate knowledge buildings.
- Grouped processing: It’s helpful for additional processing or evaluation of teams of duplicates.
Utilizing the Pandas Library
Use the pandas’ library in Python for knowledge manipulation and evaluation. We are able to use the dropduplicates() perform in pandas to take away duplicates from a listing and procure the distinctive components.
- Import the pandas’ library:
import pandas as pd
- Create a pandas Collection from the record:
my_list = [1, 2, 2, 3, 4, 4, 5]
my_series = pd.Collection(my_list)
- Use the drop_duplicates() technique:
unique_series = my_series.drop_duplicates()
- Convert again to a listing:
unique_list = record(unique_series)
Output:
[1, 2, 3, 4, 5]
Key factors:
- Protect the unique order of components: Keep the unique order of components.
- Flexibility: Customise drop_duplicates() with parameters like:
- hold: Which duplicates to maintain (“first”, “final”, or “False” for all).
- subset: Columns to think about for duplicate identification (for DataFrames).
- DataFrame dealing with: Use DataFrame.drop_duplicates() for duplicate removing in DataFrames.
Different utilizing distinctive():
unique_list = my_series.distinctive() # Additionally returns a NumPy array
Conclusion
On this weblog, we explored 7 methods to take away duplicates from a listing in Python. Every technique provides benefits and uses them primarily based on the particular necessities of the duty. By understanding these strategies, Python builders can effectively deal with duplicate components in lists and optimize their code for higher efficiency. Whether or not it’s utilizing set(), record comprehension, filter(), OrderedDict, Counter, itertools. group by (), or the panda’s library, Python offers quite a lot of instruments to sort out the widespread drawback of eradicating duplicates from a listing.
Key Takeaways
- Acknowledge that Python provides a variety of instruments, reminiscent of set(), record comprehension, filter(), OrderedDict, Counter, itertools.groupby(), and pandas, for dealing with duplicate components in lists.
- Perceive that every technique has its strengths and issues, permitting builders to decide on essentially the most appropriate method primarily based on the particular necessities of their process.
- Acquire insights into optimizing code for higher efficiency when working with lists and grasp the significance of choosing the proper technique relying on the scale and traits of the record.
- Develop problem-solving abilities by studying totally different methods, offering Python builders with versatile instruments to effectively deal with the widespread problem of eradicating duplicates from lists.
If you wish to be taught Python from scratch. Then, enroll at no cost for this course.
Often Requested Questions
A. Use the set()
constructor to get rid of duplicates in a listing: unique_list = record(set(original_list))
.
Alternatively, make use of a listing comprehension: unique_list = [x for i, x in enumerate(original_list) if x not in original_list[:i]]
.
A. The quickest approach to take away duplicates in Python is by utilizing the set()
constructor: unique_list = record(set(original_list))
.
A. To effectively take away duplicates from a listing in Python whereas preserving the unique order, you should utilize the collections.OrderedDict
method:
from collections import OrderedDict
unique_list = record(OrderedDict.fromkeys(original_list))
A. To extract duplicates from a listing in Python, you should utilize the next method:
original_list = [1, 2, 2, 3, 4, 4, 5]
duplicates = [item for item in set(original_list) if original_list.count(item) > 1]
This record comprehension creates a brand new record (duplicates
) containing gadgets that seem greater than as soon as within the authentic record whereas utilizing a set to make sure distinctive values.
Associated
[ad_2]