
[ad_1]
In case you’re new to Arduino and connecting Arduino to the web, the method might sound difficult at first. Many newbies face challenges on the subject of web connectivity whereas coding with Arduino. With no user-friendly interface and built-in debugging instruments, it may be tough to establish and rectify errors. On this article, we’ll present a complete information on connecting your Arduino board to the web, together with the required code.
After I first started enjoying round with my Arduino Uno, I used to be very pleased with making LEDs blink and sensors beep. However I quickly realized that the true potential of an Arduino can solely be achieved when you join it to the web.
You possibly can examine the modern Arduino Initiatives.
Just about any intermediate to superior stage Arduino undertaking would require you to connect with the web for varied causes, be it logging knowledge on the cloud corresponding to climate knowledge, or passing on real-time instructions to your machine remotely corresponding to through an app in your cellphone.
On this tutorial, we’re going to take a look at find out how to join your Arduino machine to the web comprehensively. All the pieces—from the {hardware} to the circuit and code—will likely be coated.
Connecting Arduino to the Web with Ethernet
The primary possibility for connecting your Arduino to the web is through an Ethernet cable. If you’re utilizing an Arduino board that comes with a built-in Ethernet port corresponding to an Arduino Yún, you possibly can skip the ‘{Hardware} necessities’ part and the circuit design description given under. Simply join the Ethernet cable to your machine and begin coding.
Nevertheless, if, like most individuals, you personal a less complicated model of Arduino corresponding to Arduino Uno that doesn’t have a built-in Ethernet port, you’ll have to purchase a separate machine, known as an Ethernet defend, to connect to your Arduino.
{Hardware} Necessities
You’ll require an Ethernet cable with the web, an Arduino Uno board, and an
Ethernet Protect. Right here’s find out how to join them:
- Join the Ethernet defend on prime of the Arduino Uno.
- Join the Ethernet cable to the Ethernet defend.
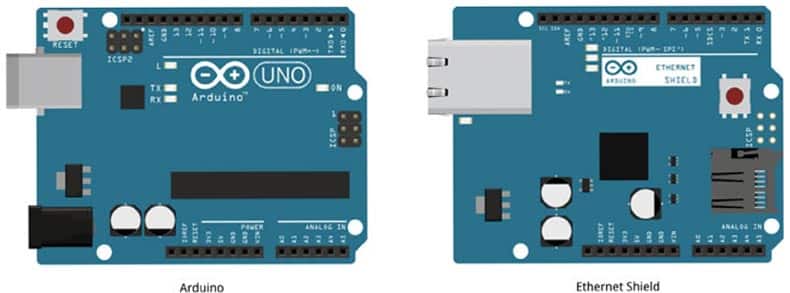
Fig. 1 exhibits Arduino Uno and its Ethernet defend. After connecting the 2, your Arduino Uno ought to appear like the one in Fig. 2.
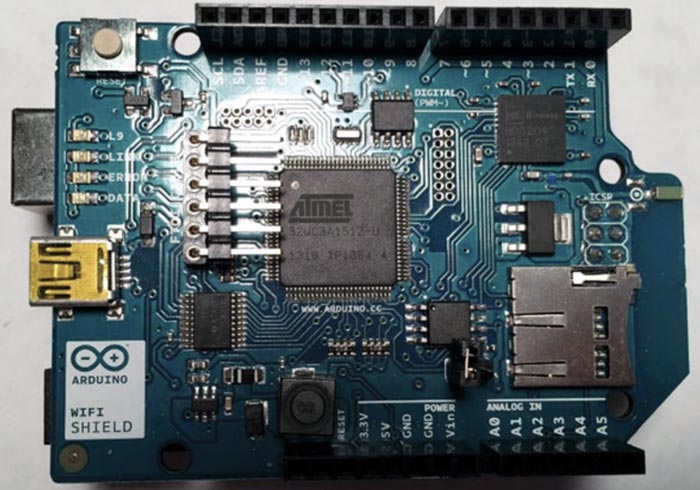
Arduino Code for Connecting to the Web through Ethernet
Earlier than getting began with the code for connecting Arduino to the web, we have to embrace an exterior library to be used in this system. This library will assist us set up a connection and ship/obtain knowledge over the web.
Notice: This library ought to come preinstalled together with your IDE. If for some cause you run into errors, please obtain this library from the official GitHub repository of the Arduino Venture.
#embrace <Ethernet.h>
Within the subsequent step, we will likely be defining some constants and variables required for this program.
First, we’d like the MAC handle. That is typically printed on a sticker on the Ethernet defend. Subsequent, we have to outline a static IP handle.
Ensure that the IP handle that you’re including isn’t being utilized by some other consumer. Lastly, we will outline the EthernetClient variable.
byte mac[] = { oxDE, oxAD, oxBE, oxEF,
oxFE, oxED };
IPAddress staticIP(10, 0, 0, 20);
EthernetClient shopper;
We will now write the strategy for connecting to the web. There are two steps right here. First, we attempt to get an IP through the DHCP (dynamic host configuration protocol), i.e., attempt to fetch your dynamic IP. If that step fails, then we fall again to the static IP that we now have outlined above.
void connectToInternet()
{
// Step 1 - Strive connecting
with DHCP
If (Ethernet.start(mac) == 0)
{
Serial.print(“[ERROR]
Failed to attach through DHCP”);
Ethernet.start(mac,
staticIP); // Join through static IP
outlined earlier
}
// Add a delay for initialization
delay(1000);
Serial.println(“[INFO] Connection
Profitable”);
Serial.print(“”);
printConnectionInformation(); // Customized
methodology
Serial.print
ln(“---------------------------------”);
Serial.println(“”);
}
As you possibly can see within the code above, we used a customized methodology printConnectionInformation() for displaying the connection info. So allow us to go forward and write the code for that.
void printConnectionInformation()
{
// Print IP Handle
Serial.print(“[INFO] IP Handle: ”);
Serial.println(Ethernet.localIP());
// Print Subnet Masks
Serial.print(“[INFO] Subnet Masks: ”);
Serial.println(Ethernet.
subnetMask());
// Print Gateway
Serial.print(“[INFO] Gateway: ”);
Serial.println(Ethernet.gatewayIP());
// Print DNS
Serial.print(“[INFO] DNS: ”);
Serial.println(Ethernet.
dnsServerIP());
}
Lastly, we will write the usual features for this system, i.e. setup() and loop().
void setup()
{
Serial.start(9600);
// Connect with the web
connectToInternet();
}
void loop()
{
// Nothing a lot to do right here.
}
If every part checks out, you have to be seeing an output just like Fig. 3 on the serial monitor window.
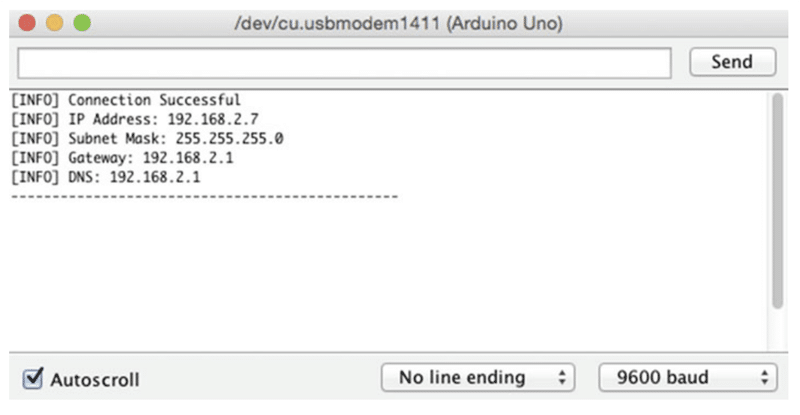
Connecting Arduino to the Web through Wi-Fi
The second possibility is to attach your Arduino to the web wirelessly through Wi-Fi. If, like most individuals, you personal an Arduino Uno or some other Arduino board that doesn’t have a built-in Wi-Fi functionality, you’ll have to purchase a wi-fi defend individually, just like the Ethernet defend.
In case you personal an Arduino Yún or some other Arduino board with built-in wi-fi functionality, you possibly can skip the ‘{Hardware} necessities’ and the outline on the following web page and get began with the code straight away.
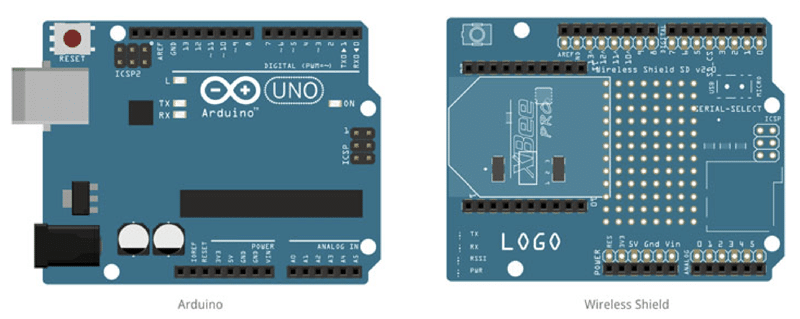
{Hardware} Necessities
You’ll need an Arduino Uno and a wi-fi defend. Right here’s find out how to join them:
- Join the wi-fi defend on prime of the Arduino Uno.
- Join the Arduino Uno to your laptop through the USB port.
Fig. 4 exhibits the Arduino Uno and the wi-fi defend. If every part is linked correctly, your Arduino Uno board ought to appear like the one in Fig. 5.
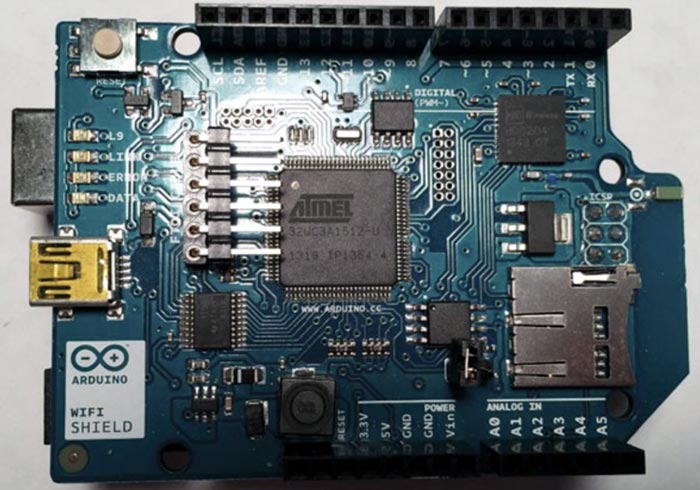
Arduino Code for Connecting to the Web through Wi-Fi
When connecting to the web through Ethernet, we used the exterior library. Equally, for connecting to the web wirelessly, we will be utilizing the exterior library.
Notice: This library ought to come preinstalled together with your IDE. If for some cause you run into errors, please obtain this library from the official GitHub repository of the Arduino Venture.
#embrace <SPI.h>
#embrace <WiFi.h>
Subsequent, we will outline the constants and variables required for connecting wirelessly. For connecting with the Wi-Fi, we require the ssid and password of the Wi-Fi that we will be utilizing. We will additionally create a WiFiClient variable for connecting to the web.
char ssid[] = “Write WiFi SSID right here”;
char move[] = “Write WiFi password
right here”;
int keyIndex - 0;
int standing = WL_IDLE_STATUS;
WiFiClient shopper;
Now we will outline some customized strategies for connecting and sending/receiving knowledge over the Web. First, allow us to create the strategy connectToInternet() for connecting with the Web.
void connectToInternet()
{
standing = WiFi.standing();
if (standing == WL_NO_SHIELD)
{
Serial.println(“[ERROR] WiFi Protect
Not Current”);
whereas (true);
}
whereas ( standing != WL_CONNECTED)
{
Serial.print(“[INFO] Trying
Connection - WPA SSID: ”);
Serial.println(ssid);
standing = WiFi.start(ssid, move);
}
Serial.print(“[INFO] Connection
Profitable”);
Serial.print(“”);
printConnectionInformation();
Serial.print
ln(“-------------------------”);
Serial.println(“”);
}
As you possibly can see within the code above, we now have known as the customized methodology printConnectionInformation() to show the details about our Wi-Fi connection. So allow us to go forward and write that methodology:
void printConnectionInformation()
{
Serial.print(“[INFO] SSID: ”);
Serial.println(WiFi.SSID());
// Print Router’s MAC handle
byte bssid[6];
WiFi.BSSID(bssid);
Serial.print(“[INFO] BSSID: ”);
Serial.print(bssid[5], HEX);
Serial.print(“:”);
Serial.print(bssid[4], HEX);
Serial.print(“:”);
Serial.print(bssid[3], HEX);
Serial.print(“:”);
Serial.print(bssid[2], HEX);
Serial.print(“:”);
Serial.print(bssid[1], HEX);
Serial.print(“:”);
Serial.println(bssid[0], HEX);
// Print Sign Power
lengthy rssi = WiFi.RSSI();
Serial.print(“[INFO] Sign Power
(RSSI) : ”);
Serial.println(rssi);
// Print Encryption sort
byte encryption = WiFi.encryption
Sort();
Serial.print(“[INFO] Encryption Sort
: ”);
Serial.println(encryption, HEX);
// Print WiFi Protect’s IP handle
IPAddress ip = WiFi.localIP();
Serial.print(“[INFO] IP Handle : ”);
Serial.println(ip);
// Print MAC handle
byte mac[6];
WiFi.macAddress(mac);
Serial.print(“[INFO] MAC Handle: ”);
Serial.print(mac[5], HEX);
Serial.print(“:”);
Serial.print(mac[4], HEX);
Serial.print(“:”);
Serial.print(mac[3], HEX);
Serial.print(“:”);
Serial.print(mac[2], HEX);
Serial.print(“:”);
Serial.print(mac[1], HEX);
Serial.print(“:”);
Serial.println(mac[0], HEX);
}
Lastly, allow us to write down the usual features, i.e. setup() and loop():
void setup()
{
Serial.start(9600);
// Connect with the web
connectToInternet();
}
void loop()
{
// Nothing to do right here
}
Hurray! We’re carried out with the Arduino code for connecting to the web through Wi-Fi. In case there aren’t any errors, the output in your serial monitor window ought to appear like the output in Fig. 6.
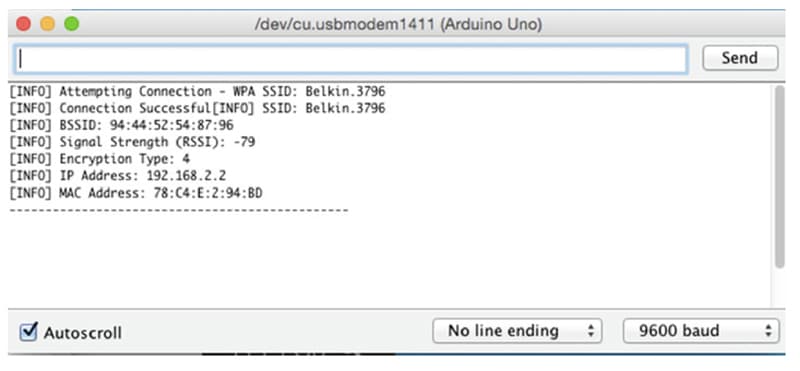
This text was first revealed within the December 2022 concern of Open Supply For You journal.
Mir H.S. Quadri is a analysis analyst with a specialization in synthetic intelligence and machine studying. He’s the founding father of Arkinfo, which focuses on the analysis and growth of tech merchandise utilizing new-age applied sciences. He shares a deep love for evaluation of technological tendencies and understanding their implications. Being a FOSS fanatic, he has contributed to a number of open-source tasks
[ad_2]